Basic JavaScript Calculator Source Code | HTML, CSS
AUTHOR: | meruem44 |
---|---|
VIEWS TOTAL: | 126 views |
OFFICIAL PAGE: | Go to website |
LAST UPDATE: | November 10, 2020 |
LICENSE: | MIT |
Preview:
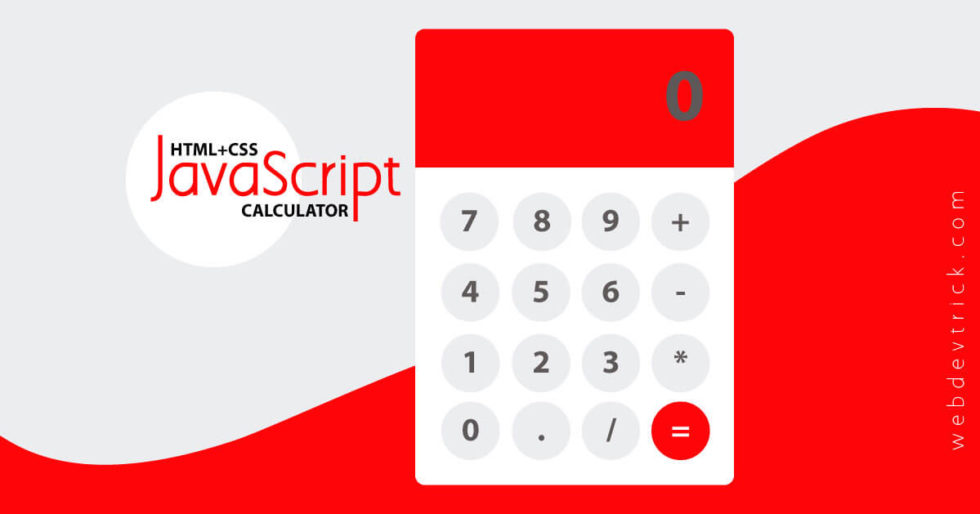
Description:
Basic JavaScript Calculator Source Code Given Here Below.
Copy these codes and save it with the name that is given here. use can use IDE as well as notepad in windows. I would recommend that first understand this code and then use it anywhere. So, if you have to make it yourself, you do not have to copy the code repeatedly.
I had been created in 2 separate files So that the code does not get long. Generate two files first “calculator.html” second “style.css”
Preview
- If you are using a phone click on Result for preview
How to use it:
1.HTML
<html>
<head>
<meta charset="utf-8">
<title>JavaSctipt Calculator | Web Dev Trick</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="container">
<form name="form">
<div class="display">
<input type="text" placeholder="0" name="displayResult" />
</div>
<div class="buttons">
<div class="row">
<input type="button" name="b7" value="7" onClick="calcNumbers(b7.value)">
<input type="button" name="b8" value="8" onClick="calcNumbers(b8.value)">
<input type="button" name="b9" value="9" onClick="calcNumbers(b9.value)">
<input type="button" name="addb" value="+" onClick="calcNumbers(addb.value)">
</div>
<div class="row">
<input type="button" name="b4" value="4" onClick="calcNumbers(b4.value)">
<input type="button" name="b5" value="5" onClick="calcNumbers(b5.value)">
<input type="button" name="b6" value="6" onClick="calcNumbers(b6.value)">
<input type="button" name="subb" value="-" onClick="calcNumbers(subb.value)">
</div>
<div class="row">
<input type="button" name="b1" value="1" onClick="calcNumbers(b1.value)">
<input type="button" name="b2" value="2" onClick="calcNumbers(b2.value)">
<input type="button" name="b3" value="3" onClick="calcNumbers(b3.value)">
<input type="button" name="mulb" value="*" onClick="calcNumbers(mulb.value)">
</div>
<div class="row">
<input type="button" name="b0" value="0" onClick="calcNumbers(b0.value)">
<input type="button" name="potb" value="." onClick="calcNumbers(potb.value)">
<input type="button" name="divb" value="/" onClick="calcNumbers(divb.value)">
<input type="button" class="red" value="=" onClick="displayResult.value=eval(displayResult.value)">
</div>
</div>
</form>
</div>
</body>
</html>
2. CSS
/**
Code By Web Dev Trick ( https://webdevtrick.com )
For More Source Code Visit Our Blog ( https://webdevtrick.com )
**/
body, html {
background: #ECEDEF;
margin: 0;
padding: 0;
}
.container {
position: fixed;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
background: #fff;
box-shadow: 0 4px 8px 0 rgba(0,0,0,0.2), 0 6px 20px 0 rgba(0,0,0,0.2);
border-radius: 14px;
padding-bottom: 20px;
width: 320px;
}
.display {
width: 100%;
height: 60px;
padding: 40px 0;
background: #FF0509;
border-top-left-radius: 14px;
border-top-right-radius: 14px;
}
.buttons {
padding: 20px 20px 0 20px;
}
.row {
width: 280px;
float: left;
}
input[type=button] {
width: 60px;
height: 60px;
float: left;
padding: 0;
margin: 5px;
box-sizing: border-box;
background: #ecedef;
border: none;
font-size: 30px;
line-height: 30px;
border-radius: 50%;
font-weight: 700;
color: #5E5858;
cursor: pointer;
}
input[type=text] {
width: 270px;
height: 60px;
float: left;
padding: 0;
box-sizing: border-box;
border: none;
background: none;
color: #ffffff;
text-align: right;
font-weight: 700;
font-size: 60px;
line-height: 60px;
margin: 0 25px;
}
.red {
background: #FF0509 !important;
color: #ffffff !important;
}
How to use it:
3.js
function calcNumbers(result){
form.displayResult.value=form.displayResult.value+result;
}
3.js
function calcNumbers(result){ form.displayResult.value=form.displayResult.value+result; }